On This Page
In this challenge, you will develop one of the main react applications for beginners. It'll help you to learn many Javascript and React concepts. This challenge differs slightly from Word and Senteces Counter and Password Generator because you need to fetch data from json-server
using Axios
.
The best part of these challenges is that they have a built-in UI, and your role is to put logic in them. So, keep an eye on every minor detail to complete the challenge.
UI and Features Suggestions for React Random Quote Application
I have kept the app in a simpler version. You can make this app better by adding more features. The more you put in the effort, the more it'll become an asset for your portfolio or can be launched as a passion project.
What can you do more?
- You can add categories, and the user can choose what kind of quote he wants to read from their favorite type.
- You can also add another feature to allow users to favorite the quote, save it in local storage, or build a Nodejs API.
- Right now, you can only view one quote at a time. If you like, you can show multiple quotes in a grid and categories of quotes at the top or bottom of the page.
Which skills are going to test?
- How to fetch API in react using axios
- How
useState
anduseEffect
work - How to share quotes on social media
- You can implement logic in Javascript
How To Start the Random Quote Challenge
If you have decided to take this challenge, so here are the steps to start this ReactJS challenge:
- Fork Reactjs Coding Challenges GitHub repo
- Go to
random-quote-generator
folder usingcd random-quote-generator
via terminal - Run npm install or yarn to install dependencies
- Run the
json-server
using the commandjson-server --watch db.json --port 4000
in terminal (you can change the port too as per your need) - Run the react server using
npm start
, you will then see the below screen on your local host
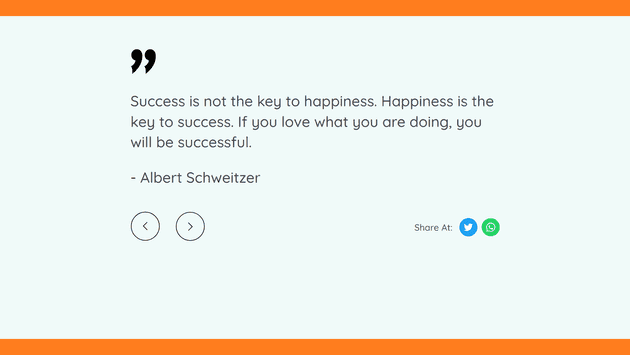
Final Result
This is the live link to the fully functional App. Try yourself to understand the working.
User Stories for Random Quote Application Challenge
- As soon the app loads, you must fetch quotes from the API using
axios
, randomize the API data and save all quotes inquoteList
local state - As soon the app loads the app will show the user the first quote stored in the
quoteList
array - The quote will appear with quote text and author name as well (see the demo app)
- The user cannot click the back/previous on the first load. Make it disabled at the beginning.
- The app should show next quote from the local state (
quoteList
) when the user clicks the next button - When you select the previous button, a lastly showed random quote will be displayed. The app will show the quote from
quoteList
- Once the user generates all quotes, the local state (
quoteList
) will be reset (empty), and repeat point 1 - It may take a second or less to fetch data from the API. So when there is no data, show the "Loading…" text in the UI instead of an empty screen. After fetching data, replace it with API data (quote and author)
- Users can share quotes with the author's name with his WhatsApp contacts and Twitter profile
- Use typescript, as you can add more features to the application with intellicence and less chances of error
- The browser console should not have an error
Extra Marks
- Destructure the data wherever needed
- Create a separate file for utils functions (you can name it
utils.ts
), in this challenge we need one util Javascript function for randomizing the array - Create a custom hook
useQuoteFetcher
for fetching data, and for all the business logic,API
URL will be passed as param, API URL in our case islocalhost:4000/quotes
(you can change the port if you need)
Hints and Resources
- Learn how json server works
- Use
math.random()
to randomize the data - Understand how to fetch requests with
axios
- You need to keep track of current quote index for next and previous quote
- To share on whatsapp use url
https://wa.me/?text=...
and for twitterhttps://twitter.com/intent/tweet?text=...
with encodeURIComponent - Fullstack React with TypeScript is the complete guide to using TypeScript with React. Learn TypeScript patterns with React additional ecosystem advice (testing, redux, SSR) by building several apps including a Trello clone, a Medium-like website, testing with a digital-item e-comm app, and more!
Working Solution For Random Quote Application
For suggestions and collaboration, you can send me an email at [email protected] or can reach out to me on LinkedIn or Twitter.
Important!
You can also subscribe to Codevertiser Magazine Newsletter and get the latest coding challenges delivered straight to your inbox