When working on an application with a timer, converting time can be a headache. Imagine you are given the time in seconds and need to display it in hours:minutes:seconds format. How do you do it?
It is a common problem for developers who deal with time-sensitive applications, and it can be frustrating to figure out. In this blog, I will guide you through converting seconds into the hours:minutes:seconds time format, and you'll also get free resources to practice at the end of it.
Don't let time conversion slow you down!
Use cases: Convert Seconds to Hours and Minutes Function
The conversion of seconds to hours and minutes is a critical function with a wide range of use cases. Here are some examples of situations where this function can come in handy:
-
When tracking time for tasks, you can display the time in hours and minutes. You can record time in a more user-friendly format.
-
In scheduling applications, it ensures the time is displayed correctly, and the schedule is easily read.
-
Clocks and timers must often display time in the hours and minutes format.
-
It can also be used in a quiz app or Pomodoro clock.
How to Convert Seconds into Minutes and Hours?
You can convert seconds into minutes and hours in just some simple steps. I have divided the process into four steps so you can understand it with ease. Also, you can implement what you think you need. So let’s get started.
Step 01: Create Base Function:
First, we are going to write the base structure of the function convertSeconds
. It will take seconds as a number parameter and will return a string.
const convertSeconds = (seconds: number): string => {return ''}
Step 02: Calculate Minutes and Hours
Once you have created the base function, you will need to convert seconds into minutes and hours. Here’s how can you do it:
1. Javascript Change Seconds to Hours
As you know one hour is equal to 3600 seconds, so divide the total seconds by 3600 to get the number of hours. It’ll sometimes have the result in decimal, but we need it in whole numbers. So, we’ll use Math.floor
to round down the value.
const hours = Math.floor(seconds / 3600)
2. Javascript Change Seconds to Minutes
After calculating the exact hours, now we will convert the remaining seconds into minutes. For this, we’ll use the reminder technique. Here we’ll first get the reminder of hours.
const minutes = Math.floor((seconds % 3600) / 60)
seconds % 3600
will return the remaining seconds that are less than one hour. Now, divide it by 60 as one minute has 60 seconds.
const convertSeconds = (seconds: number): string => {const hours = Math.floor(seconds / 3600)const minutes = Math.floor((seconds % 3600) / 60)return `${hours} hours : ${minutes} minutes`}const result = convertSeconds(3800)console.log(result) // 1 hours : 3 minutes
Step 03: Handling the Conditions
What if seconds are less than an hour? In this case, I will prefer to show only minutes like 36 minutes not 0 hours: 36 minutes.
const convertSeconds = (seconds: number): string => {const hours = Math.floor(seconds / 3600)const minutes = Math.floor((seconds % 3600) / 60)if (hours > 0) {return `${hours} hours : ${minutes} minutes`} else {return `${minutes} minutes`}}const result = convertSeconds(2200)console.log(result) // 36 minutesconvertSeconds(3800) // 1 hours : 3 minutes
In the above condition, you have seen the result is 1 hour. You can notice here that hours are shown with ‘s.’ We have to set this too. If hour is greater than 1 will return hours (with s) otherwise hour, same goes for seconds.
const convertSeconds = (seconds: number): string => {const hours = Math.floor(seconds / 3600)const minutes = Math.floor((seconds % 3600) / 60)if (hours > 0) {return `${hours} hour${hours > 1 ? 's' : ''} : ${minutes} minute${minutes > 1 ? 's' : ''}`} else {return `${minutes} minute${minutes > 1 ? 's' : ''}`}}const result1 = convertSeconds(2200) // 36 minutesconst result2 = convertSeconds(3950) // 1 hour : 5 minutesconst result3 = convertSeconds(9004) // 2 hours : 30 minutesconst result4 = convertSeconds(61) // 1 minuteconst result5 = convertSeconds(3601) // 1 hour : 0 minute
Till now we have converted seconds into hours and minutes but it is not displaying the remaining seconds. For example, 3950 seconds can be converted to 1 hour : 5 minutes : 50 seconds, but our result is not displaying seconds. To show the remaining seconds, we have to refactor and format the code to avoid many if-else statements.
const convertSeconds = (seconds: number): string => {const hours = Math.floor(seconds / 3600)const minutes = Math.floor((seconds % 3600) / 60)const remainingSeconds = seconds % 60const hourString = hours > 0 ? `${hours} hour${hours > 1 ? 's' : ''}` : ''const minuteString = minutes > 0 ? `${minutes} minute${minutes > 1 ? 's' : ''}` : ''const secondString =remainingSeconds > 0 ? `${remainingSeconds} second${remainingSeconds > 1 ? 's' : ''}` : ''return `${hourString} : ${minuteString} : ${secondString}`}const result1 = convertSeconds(2200) // : 36 minutes : 40 secondsconst result2 = convertSeconds(3950) // 1 hour : 5 minutes : 50 secondsconst result3 = convertSeconds(9004) // 2 hours : 30 minutes : 4 secondsconst result4 = convertSeconds(61) // : 1 minute : 1 secondconst result5 = convertSeconds(3601) // 1 hour : : 1 second
Step 04: Dealing with Edge Cases and Conditions
Finally, we are at the last step of our time conversion function. The above result shows space or empty if hours, minute, or seconds is 0 or missing, and display like : 36 minutes : 40 seconds. We need to fix this too.
const convertSeconds = (seconds: number): string => {const hours = Math.floor(seconds / 3600)const minutes = Math.floor((seconds % 3600) / 60)const remainingSeconds = seconds % 60const hourString = hours > 0 ? `${hours} hour${hours > 1 ? 's' : ''}` : ''const minuteString = minutes > 0 ? `${minutes} minute${minutes > 1 ? 's' : ''}` : ''const secondString =remainingSeconds > 0 ? `${remainingSeconds} second${remainingSeconds > 1 ? 's' : ''}` : ''if (hours > 0) {return `${hourString} : ${minuteString || '0 minute'} ${secondString && `: ${secondString}`}`} else if (!hours && minutes > 0) {return `${minuteString} ${secondString && `: ${secondString}`}`}return secondString}const result1 = convertSeconds(2200) // 36 minutes : 40 secondsconst result2 = convertSeconds(3600) // 1 hour : 0 minuteconst result3 = convertSeconds(9004) // 2 hours : 30 minutes : 4 secondsconst result4 = convertSeconds(61) // 1 minute : 1 secondconst result5 = convertSeconds(3601) // 1 hour : 0 minute : 1 secondconst result6 = convertSeconds(180) // 3 minutesconst result7 = convertSeconds(50) // 50 seconds
If you want to improve this code or change the time format, go ahead and also let me know what you created.
Apply What You've Learned
It is excellent if you have reached out so far and learned how to convert time using JavaScript. To further enhance your logic, you can implement what you have learned in this article in the following ways:
- I built a quiz app in ReactJS without a timer in 6 steps to create a quiz app article. You can incorporate timer in the top right corner of the quiz app. It'll make it more functional and classier.
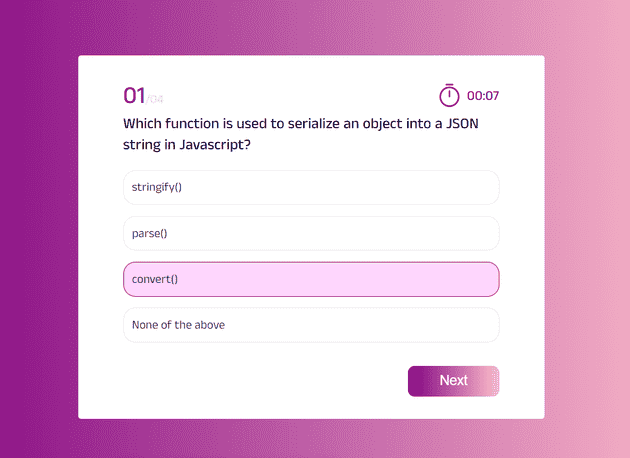
- In one of the freecodecamp projects, you are challenged to build a Pomodoro Clock, for pomodoro clock you need to deal with a timer, and you can apply the above learning to it.
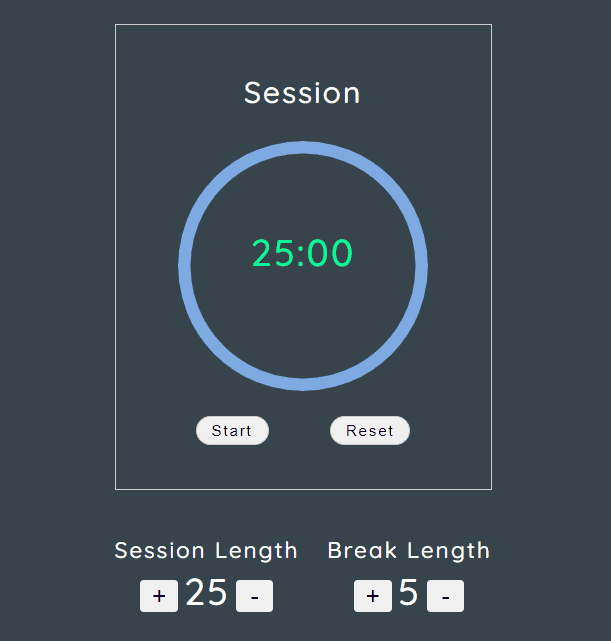