On This Page
- # What you will learn by building the React Password Generator App
- # How to start the project
- # User Stories for React Password Generator Challenge
- # 1. Initial Password Display and Regenerate Password
- # 2. Copy Password to Clipboard
- # 3. Checkbox Functionality
- # 4. Automatic Checkbox Selection
- # 5. Password Length Validation
- # 6. Password Strength Logic
- # 7. Visual Password Strength Representation
- # 8. TypeScript Integration and Console Checks
- # Hints and Resources
If you search on Codecanyon for "React Password Generator tool," you will find scripts that people are selling for $10-12 and have multiple sales.
What if you build your own password generator tool that has all the functionalities a paid tool has?
A good password generator app has three key features:
-
Creates strong passwords with uppercase, lowercase, numbers, and special characters.
-
Lets you copy the password to the clipboard.
-
Allows setting password length.
And in this challenge, you will build all three functionalities.
The good news about this free React challenge is that you don't have to invest energy in developing UI. We have already created the UI; you only need to implement the logic and make the tool functional.
Get the code
If you don't want to do the challenge, download the React Password Generator script from Gumroad for free.
What you will learn by building the React Password Generator App
React Password Generator challenge will test you in the following skills:
- Familiarity with the react
useState
anduseEffect
hook - How to randomize string characters or array elements in javascript
- How to copy to clipboard works in ReactJS
- How to conditionally change state in Reactjs using if condition and for loops
How to start the project
- Fork Reactjs Coding Challenges GitHub repo
- Go to password-generator folder using
cd password-generator
via terminal - Run npm install or yarn to install dependencies
- Run the server, you will then see the below screen on your local host
You are good to go
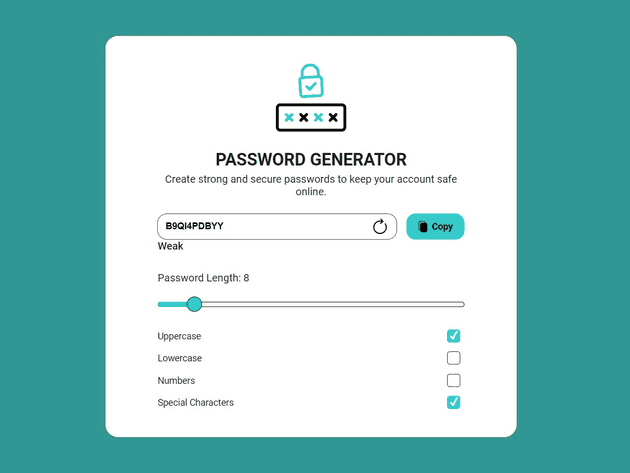
Functional Live App
You have to make the app functional by writing logic that looks like this.
User Stories for React Password Generator Challenge
1. Initial Password Display and Regenerate Password
- As soon the app loads, input will contain a random password of length eight characters
- Every time you click the refresh button (icon) it will generate a new password
2. Copy Password to Clipboard
- Clicking the copy button copies the password to the clipboard. (plugin is already installed)
- The copy button text changes to copied from copy for 1 second (refer to the demo app for clarification).
3. Checkbox Functionality
- Users will be able to check or uncheck uppercase, lowercase, number, and special characters checkboxes
- Uppercase letters: ABCDEFGHIJKLMNOPQRSTUVWXYZ
- Lowercase letters: abcdefghijklmnopqrstuvwxyz
- Numbers: 0123456789
- Special Characters: !@#$%^&*()
4. Automatic Checkbox Selection
- If all checkboxes are unchecked, the lowercase checkbox automatically becomes checked, for a better understanding play with the demo app
5. Password Length Validation
- If password length is less than 8, the password strength message (below password input) will be “Too short”, and the color will be Red
6. Password Strength Logic
- You also have to write password strength logic, password strength will show below password input (below logic will be applicable if password length is equal to or greater than 8).
- Hard: password contains at least one uppercase, one lowercase, one number, and one special character
- Medium: if one of the fields is missing in the password
- Easy: if two of the fields are missing in the password
7. Visual Password Strength Representation
- Different colors should represent the password strength; you can find color’s HEX codes in
variables.css
file and utility classes instyles.css
file- Weak: Red
- Medium: Orange
- Hard: Green
8. TypeScript Integration and Console Checks
- Ensure that all code is written using TypeScript for this React project.
- Verify there are no errors or warnings in the console.
Hints and Resources
- For password strength logic you can create an array, then run a loop, and inside the loop, look for conditions and test for generated password
- react-copy-to-clipboard npm package will help you to copy to the clipboard feature (already installed)
- Fullstack React with TypeScript is the complete guide to using TypeScript with React. Learn TypeScript patterns with React additional ecosystem advice (testing, redux, SSR) by building several apps including a Trello clone, a Medium-like website, testing with a digital-item e-comm app, and more!
Looking for an answer?
For suggestions and collaboration, you can send me an email at [email protected] or can reach out to me on LinkedIn or Twitter.
Important!
You can also subscribe to Codevertiser Magazine Newsletter and get the latest coding challenges delivered straight to your inbox