In this article, we are going to talk about the power of styled-components, by learning how can we reuse styles in styled components. There are many benefits of using styled components in react project, one of them is we can also write reusable styled components. Reusable styles make our project code clean, maintainable, and easier to refactor.
We will discuss two ways to reuse styles:
- Reusable Styled Components
- Reusable utility classes
1. Reusable Styled Components
In this section, we are going to discuss and write popular and most commonly used styled components that you can use in your project as well. We usually put these components in some common place of the project instead of some specific files. I prefer writing reusable components in Global.js
file.
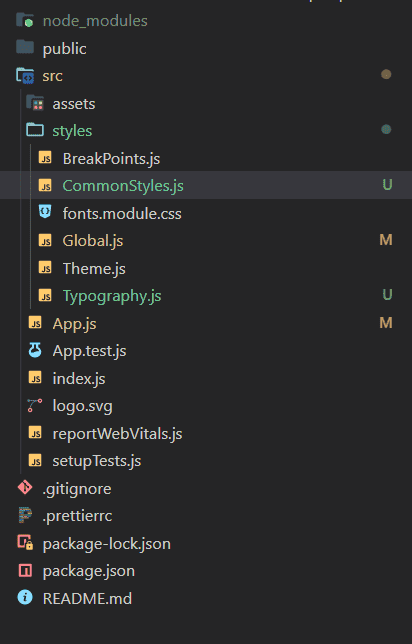
i. Container in Styled Components
The container
component of styled components is similar to the container in bootstrap, material UI, and other CSS frameworks. That is used for containing or wrapping things in a specific area.
export const Container = styled.div`width: 100%;max-width: 1360px;margin: 0 auto;padding: 0 ${({ theme }) => theme.paddings.container};${({ medium }) =>medium &&css`max-width: 1200px;`}${({ small }) =>small &&css`max-width: 1100px;`}`
ii. Grid Component in styled components
Responsiveness is an important aspect of all modern web projects, it allows our site to be flexible for viewing on multiple types of devices. We can use either grid or flex to make our site responsive.
The Grid component can be implemented by just wrapping its children inside and passing the props. If you like you can customize this component as per your need.
export const Grid = styled.div`display: grid;grid-template-columns: repeat(${({ columns }) => columns || 2}, 1fr);justify-items: ${({ end }) => end && 'end'};grid-column-gap: calc(${({ cgp }) => cgp} * 1px);${({ pt }) =>pt &&css`padding-top: calc(${pt} * 1px);`}${({ pb }) =>pb &&css`padding-bottom: calc(${pb} * 1px);`}${({ fullHeight }) =>fullHeight &&css`height: 100%;`}${({ py }) =>py &&css`padding-top: calc(${py}* 1px);padding-bottom: calc(${py}* 1px);`}`
iii. Box Component in Styled Components
The Box component makes development faster. It is used to give margin or padding to some independent component or jsx
. Let's say we have a Button
component, and we have to add margin
around it and align
it on the screen as well. Normally, we would put it in a div
and then position
that div
.
The Box Component
in React replaces this process by letting us wrap the Button component
inside the box, and passing the related props.
export const Box = styled.div`margin-top: calc(${({ mt }) => mt} * 1px);${({ flxRight }) =>flxRight &&css`display: flex;justify-content: flex-end;`}${({ smNone }) =>smNone &&css`${SmallDevicesHidden}`}`
2. Utility Classes in Styled Components
Utility classes are not like components, instead, they behave like the mixins
that we have in SCSS
.
Here, we import CSS
from styled-components, and then call utility classes inside styled components. This smoothens the development process by reducing a couple of lines of code as well as development time, you can make as many classes as you want.
i. Horizontally and vertically center an element using flexbox
export const FlxCenter = css`display: flex;justify-content: center;align-items: center;`
How to use Utility Classes
This is how we can use the above FlxCenter
utility class inside a styled components.
import styled from 'styled-components'import { device } from 'styles/BreakPoints'import { FlxCenter } from 'styles/Global'const CardStyle = styled.nav`display: flex;justify-content: space-between;@media ${device.md} {${FlxCenter}}`
ii. For absolute center
export const AbsCenter = css`top: 50%;left: 50%;transform: translate(-50%, -50%);`
iii. Hide for small devices
export const SmallDeviceShow = css`@media screen and (min-width: 600px) {display: none;}`
And that is it for styled components. Feel free to use these codes in your projects, you can access them from the GitHub Repository.
Do share this post if it helped you learn something new. You’ll also find more posts related to React and Javascript on the home page.