On This Page
When working with React, one key decision developers often face is how to organize their component files. In this article, we'll explore two popular React folder structures and naming patterns. If you're new to these concepts, this article will provide valuable insights.
Introduction
The two primary patterns we'll learn in this article are:
- Barrel Pattern
- Direct File Naming Approach
I have worked with both patterns, and both have their pros and cons, which we will discuss one by one. We will also discuss and provide solutions to the drawbacks of these patterns (if there are any).
1. Barrel Pattern:
In the Barrel pattern, which I am also using in the React Quiz App Template (I built recently with TypeScript and styled components), the biggest issue I found was navigating the files in VS Code. Let me illustrate it.
Actually, in the Barrel pattern, we structure and organize React components this way:
Create a folder and name it the same as the component name. Then create a file index.tsx
inside the folder. In case we have a style file, I will create another file inside the folder, named styled.ts
or index.scss
, depending on the CSS frameworks we are using.
Button [folder name]-- index.tsx [file name]-- style.scss [style file]
An image of the Barrel Pattern folder structure:
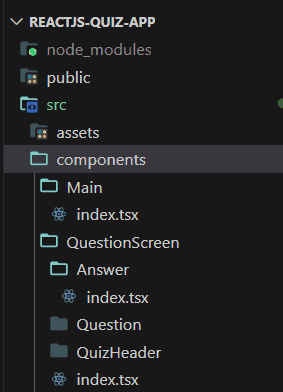
Now the problem is, each file name is index.tsx
and it's a bit hard to navigate in vs code.
An image of VS Code top tabs:

You may wonder, why I am still using this pattern?
Actually, there is one benefit of the Barrel pattern: we have shorter and more concise import paths.
For example, if I want to import a component, I will import it like this:
import Button from './components/Button'
Summary of Barrel Pattern:
Pros:
-
Shorter import paths lead to more concise and readable code.
-
Abstraction of internal component structure for cleaner folder hierarchies.
Cons:
- Potential navigation challenges in code editors due to multiple files named "index.tsx."
2. Direct File Naming Approach:
In direct file naming approach, we structure React Components this way.
Button [folder name]--Button.tsx [file name]
An image of the Direct File Naming Approach folder structure:
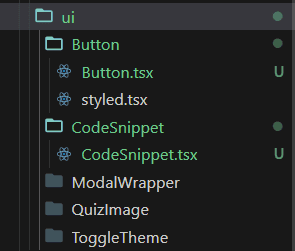
While direct file naming approach fixes the navigation problem because we have different file names for each component,

Now the problem is we have slightly longer and redundant import paths.
import Button from 'components/Button/Button'
Fixing Longer Import Paths in React
We can fix this longer import by creating another file index.tsx
in components folder. This new index.tsx
file content will look like this:
import Button from './Button'export default Button
Now in index.tsx
file we first import and then export all the files of Button Component, if you like you can name export as well in case of multiple files.
But cons of this approach is you have to create an extra file for each component you create.
Hope you get the point.
Further Reading
Read the article "How to Style a React App with Styled Components" if you want to learn more about React folder structures.
Summary Of The Article
Pros:
- Avoidance of potential navigation issues in code editors.
Cons:
- Slightly longer import paths can lead to more verbose code.
INFO
If you are a beginner and haven't worked in a large codebase, you may wonder why we are creating folders for each component.
The answer to this question is, in production, we write test and story files for each component. This folder-per-component approach simplifies code management and scalability.
Both patterns are used in real-world projects, and the choice often depends on factors such as team preferences, project size, and coding style conventions.