On This Page
Do you wonder how Memory Game logic works or want to write your own using ReactJS?
For the 5th challenge, I decided to add an interesting game: the Memory Game, I named it Memory Marvel.
This challenge is specially designed to test your JavaScript and React logics.
All these challenges take too much time to design, develop, and publish, so don’t forget to give it a star on GitHub. It will motivate me to create more free content.
How To Start React Memory Game Challenge
You just need to follow 4 simple steps to participate:
- Fork Reactjs Coding Challenges GitHub repo
- Go to
memory-marvel
folder usingcd memory-marvel
via terminal - Run npm install or yarn to install dependencies
- Run the vite server using
npm run dev
, (I used vite to create this app) you will then see the below screen on your local host
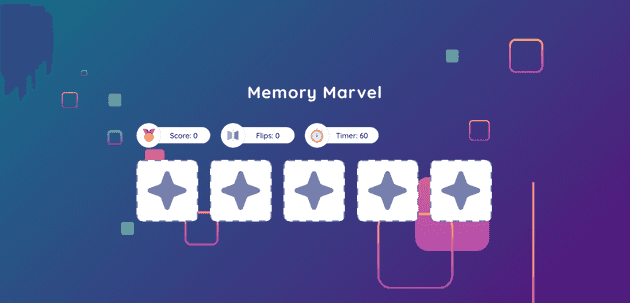
User Stories
Step #1: Set Up JavaScript Functions
- You need to write two JavaScript functions: one for duplicating cards (shapes) and the other for shuffling all cards.
Step #2: Implement Card Interaction
- User can click on any card to flip it over and reveal its content/shape.
- Conditionally add the
flipped
class in theCard
component to flip the card (the class is already styled in the CSS file). - Ensure that as a player, the cards stay flipped over if they match.
- Ensure that as a player, the cards flip back face-down if they do not match, allowing for another attempt (see the demo for clarity).
Step #3: Add Timer and Scoring System
- Implement a timer that starts counting backward when the first card is clicked.
- Display a move counter (at the top) that increments with each move.
- When cards match successfully, add 10 to the score; in case of an unmatched pair, deduct 5 from the score.
Step #4: Game Completion and Modal Display
- As a player, I want the game to end when I successfully match all the cards and modal will show up.
- If the timer reaches zero, finish the game and display the modal.
- Display the total move count and score on the modal when the game finishes.
- On clicking the refresh icon on the modal, everything will reset, and the game will restart. (see the demo for clarity).
Extra Marks: Enhance Game Configuration and Organization
- Write a logic in a way that the app can be easily configured in the future. For instance, if you wish to add game difficulty, configure the timer, or introduce a new theme.
- Create a utility file for JavaScript/TypeScript functions.
- Create a constants file for values such as initial timer settings and scores.
- Define all TypeScript types, remove any.
- Make sure there isn't any error in the console.
Further Instructions
- All icons are located in
src/config/icons.ts
. - Card data is stored in
src/data/index.ts
.
UI and Feature Suggestions for React Memory Game
- You can add a sound for a matched or incorrect move, so the user can have a more engaging experience.
- You can also add a pause and resume game option if the user needs to take a break.
- You can also add one more screen to let the user choose different difficulty levels (e.g., number of cards, card types, and match 2 or 3 cards).
Working Solution For React Memory Game
For suggestions and collaboration, you can send me an email at basit@codevertiser.com or can reach out to me on LinkedIn or X.
Important!
You can also subscribe to Codevertiser Magazine Newsletter and get the latest coding challenges delivered straight to your inbox