On This Page
I have divided this tutorial into two parts,
- Basic Setup
- Actual logic to insert data to MongoDB using nodejs
So, let’s jump into the step-by-step guide on how to import a JSON file to MongoDB Atlas using NodeJS here:
Basic Setup
Let’s begin with the basic setup of connecting NodeJS app to the MongoDB Atlas database.
Create the project
Let's make the folder upload-json-to-mongodb
after the folder has been created move to the folder via terminal and run the command.
npm init
As you've successfully created the NodeJS project, it's time to install the required dependencies.
Installing the Required Dependencies
Run the following command in the terminal to install the required dependencies on your project:
npm i express mongoose dotenv cors
Once you’re done with that, make a file named server.js
in the root directory for building the basic express server.
Create the Basic Express Server App
Now, let’s quickly create the basic express server app with the following code.
const express = require('express')const app = express()app.get('/', (req, res) => {res.send({title: 'hello world',})})const port = process.env.PORT || 5000app.listen(port, () => console.log(`listening to port ${port}`))
Run the above code in the terminal using nodemon
. But if you currently don’t have nodemon on your system, you can install it globally using the command line:
npm install --global nodemon
At this point, if you visit localhost://5000 using the address bar in your browser, then you’ll see the result like this:
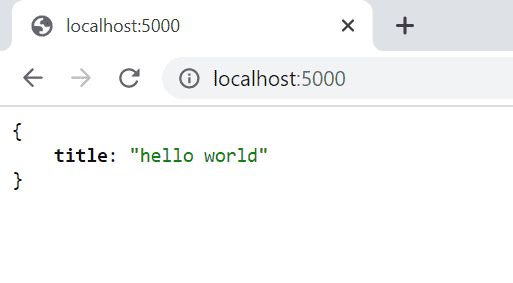
If yes, then this means that the basic express server app is active and running successfully! Now, we’ll work on the JSON file for the data upload in the MongoDB database.
Create the JSON File to Upload in MongoDB
Let’s create the JSON file that we’ll upload to the MongoDB database. Our JSON file will have the following data – as the following snippet.
;[{name: 'John Doe',position: 'Frontend Developer',experience: '2 years',},{name: 'Sara',position: 'UI/UX Designer',experience: '1.5 years',},{name: 'Mike',position: 'Backend Engineer',experience: '4 years',},]
I’ve named this file freelancer.json
, and placed this file at the root of the application.
Creating the Schema
For the schema, we create a folder named models
. Go inside this folder, and create a file with the name Freelancer.js
.
Now, write the following code in this file:
const mongoose = require('mongoose')const Schema = mongoose.Schemaconst FreelancersSchema = new Schema({name: {type: String,required: [true, 'Freelancer must have name'],trim: true,unique: true,},position: {type: String,required: [true, 'Freelancer must have position'],trim: true,},experience: {type: String,required: [true, 'Experience is required'],trim: true,},})const Freelancers = mongoose.model('Freelancer', FreelancersSchema)module.exports = Freelancers
As you can see in the above schema, you are required to input only three properties. That includes name, position, and experience.
Connecting to the MongoDB Atlas
You’ll have to use this code to setup the connection to the MongoDB database:
const express = require('express')const cors = require('cors')const mongoose = require('mongoose')const dotenv = require('dotenv')dotenv.config({ path: './config.env' })const mongoURI = process.env.MONGODB_URLconst app = express()app.use(express.json())app.use(cors())mongoose.connect(mongoURI).then(() => console.log('db connected'))app.get('/', (req, res) => {res.send({title: 'hello world',})})const PORT = process.env.PORT || 5000app.listen(PORT, () => console.log(`listening to port ${PORT}`))
Just to let you know that MongoDB URI is hidden in the config.env
file.
Till here, you did all the basic steps to connect your Node.JS application with the MongoDB Atlas database. So now, you can move to the next section to learn the actual logic for importing the JSON file to MongoDB Atlas Using NodeJS.
Actual Logic
In this part of the tutorial, you are going to work on the logic to insert the data file to MongoDB.
Here are the following simplified steps to get it done in just a few minutes:
Read the JSON File Synchronously
To read the file, let’s use the readFileSync
method. This takes two parameters that are the file name and the character encoding.
const fs = require('fs')const data = JSON.parse(fs.readFileSync('./freelancer.json', 'utf-8'))console.log(data)
As you may have noticed, I’ve used the NodeJS built-in fs
module's readFileSync
method to read the file synchronously. And in our case, it works perfectly!
After executing the above code, you can see the following log in the terminal:
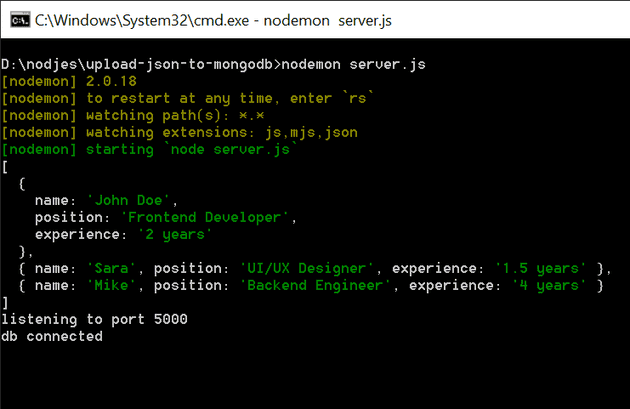
Finally, we’ll move to the last step where we will write a function to insert our JSON file to MongoDB.
Upload the JSON File to the MongoDB Server
Now to upload the file we need to write a function that will import data to the server.
we are using mongoose
create method and passing our JSON data to it, which we read above.
const importData = async () => {try {await Freelancers.create(data)console.log('data successfully imported')// to exit the processprocess.exit()} catch (error) {console.log('error', error)}}
In the end, we call the importData
function.
Lastly, you can go to the MongoDB Atlas or Compass to check whether the JSON data has been successfully uploaded or not. If yes, then you will see the following collection:
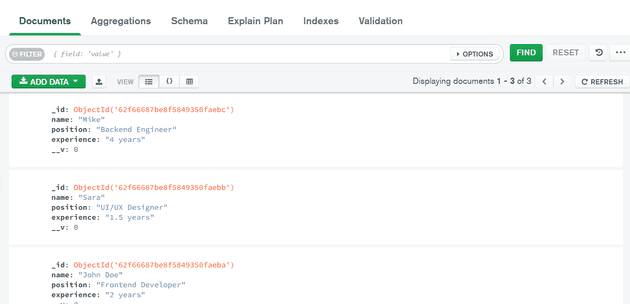
I hope you’ve learned a lot and got the expected results from my tutorial. Happy Coding!
Complete Script to Import JSON File to MongoDB Atlas Using NodeJS
For your convenience, I’m posting the whole script of this tutorial in the section below:
const fs = require('fs')const express = require('express')const cors = require('cors')const mongoose = require('mongoose')const dotenv = require('dotenv')const Freelancers = require('./models/Freelancers')dotenv.config({ path: './config.env' })const mongoURI = process.env.MONGODB_URLconst app = express()app.use(express.json())app.use(cors())// connect to mongodbmongoose.connect(mongoURI).then(() => console.log('db connected'))app.get('/', (req, res) => {res.send({title: 'hello world',})})const data = JSON.parse(fs.readFileSync('./freelancer.json', 'utf-8'))console.log(data)// import data to MongoDBconst importData = async () => {try {await Freelancers.create(data)console.log('data successfully imported')// to exit the processprocess.exit()} catch (error) {console.log('error', error)}}// importData()const PORT = process.env.PORT || 5000app.listen(PORT, () => console.log(`listening to port ${PORT}`))
There is always more we can do to optimize this script, like by calling the importData
function through the terminal, but let’s leave that for another day!
Did you learn something new from this tutorial? Or do you have more ideas to talk about? Share here with the community.