On This Page
In this article, we are going to learn how to update an object property inside an array in javascript.
We will be using javascript higher order map method, that returns a new array, which means we are not mutating the original array (data).
To use the logic anywhere in app I turned it into a function, that takes 3 parameters.
- The list (array) we want to update
- id of the object we want to update
- updated data (object)
function update(arr, id, updatedData) {return arr.map((item) => (item.id === id ? { ...item, ...updatedData } : item))}const arr = [{ id: '01', name: 'John', email: 'john@email.com' },{ id: '02', name: 'Sara', email: 'sara@email.com' },{ id: '03', name: 'Michael', email: 'michael@email.com' },]const id = '01'const updatedData = { email: 'john1@email.com' }const result = update(arr, id, updatedData)console.log(result)
This function can be used to update any kind of UI in javascript or javascript related framework, like React, Vue, Angular, etc.
Let's break down the answer into pieces.
1. Loop through Array via map method
According to MDN docs:
The
map()
method creates a new array populated with the results of calling a provided function on every element in the calling array.
map
method takes callback
in which we are writing a condition item.id === id
if this condition is fulfilled, return and update only that object, and leave the rest of the elements of an array as it is.
2. Updating object using Spread Operator
For updating object, we are using javascript spread operator. We say, keep the old data, and add the updated data to old data {...item, ...updatedData}
That’s all!
Do you wonder why old email is replaced by new email?
Can Javascript Object have duplicate keys?
No, this is how the javascript object works, it can’t have duplicate keys (keys with the same name), it will override old values with the latest one.
Example
const obj = {id: '01',name: 'John',id: '02',}console.log(obj) // output: {id: '02', name: 'John'}
Last but not least,
Let's verify if our original array is mutated or not just by console.log
the array after result.
....const arr = [{ id: '01', name: 'John', email: 'john@email.com' },{ id: '02', name: 'Sara', email: 'sara@email.com' },{ id: '03', name: 'Michael', email: 'michael@email.com' },]const id = '01'const updatedData = { email: 'john1@email.com' }const result = update(arr, id, updatedData)console.log('result', result)console.log('original array', arr)
Output
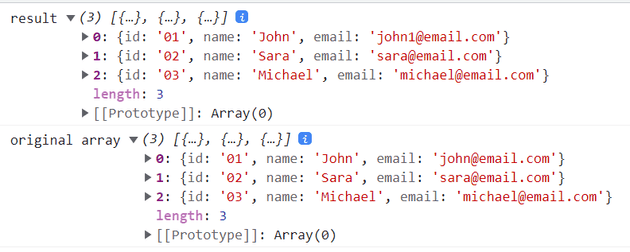
If we check the console we can see our original array data is not mutated and same as it was before.
We will be writing a TypeScript version of the function that updates an object in an array, as TypeScript has become increasingly popular in the market.
Update Object in Array with Typescript
interface Person {id: stringname: stringemail: string}function update(arr: Person[], id: string, updatedData: Partial<Person>): Person[] {return arr.map((item) => (item.id === id ? { ...item, ...updatedData } : item))}const person: Person[] = [{ id: '01', name: 'John', email: 'john@email.com' },{ id: '02', name: 'Sara', email: 'sara@email.com' },{ id: '03', name: 'Michael', email: 'michael@email.com' },]const id = '01'const updatedData = { email: 'john1@email.com' }const result = update(person, id, updatedData)console.log(result)
Here you may have noticed updatedData
parameter is given the type Partial<Person>
.
Partial<Person>
means that the updatedData
object can have some, but not necessarily all, of the properties that the Person object has.
This allows us to update only a subset of properties of the Person object.
If you have learned something new from this article, feel free to share it with your friends.