On This Page
If you are a beginner React developer, the first thing you should learn is to understand state and props, and how to build reusable components in React.
This challenge is all about those skills.
In this ReactJS challenge, you are provided a HTML for a simple blog app and and your task is to break it down into efficient, flexible React components using best practices. It’s a great way to practice component-based design and will enhance your skills in making clean, modular code.
Benefits of Taking This Challenge
- Hands-On Practice: Converting HTML to React components is a task real React developers do often.
- Build Reusable Components: Learn to make components that you can use in different parts of an app.
- Get Feedback: Receive tips on your code to improve and learn best practices.
- Showcase Your Work: Add this project to your portfolio to show employers your React skills.
How to Take Part
- Copy the HTML code provided below.
- Test the HTML code by creating an index.html file or using the tailwind playgroud.
- Create a React app with TypeScript using Vite.
- Configure Tailwind CSS in your project.
- Break down the HTML structure into reusable React components. Think about the structure and how you would design it to make each part reusable.
- Follow React best practices as you create components. Ensure they are reusable, follow naming conventions, and keep the structure modular.
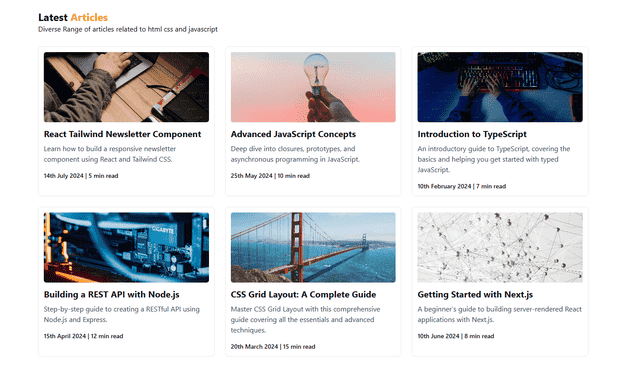
How to Submit
Want feedback on your code?
Two ways to submit your solution:
- Once you’ve converted the HTML to React components, upload the code on CodePen and share the link.
- Push your solution to a public GitHub repository and submit the repo link.
Submit Your Answer and Get Feedback.
Blog Layout HTML Structure
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8" /><meta name="viewport" content="width=device-width, initial-scale=1.0" /><script src="https://cdn.tailwindcss.com"></script><title>Blog App</title></head><body><section class="mx-auto max-w-7xl px-3"><div class="my-7"><h2 class="text-2xl font-bold text-[#161616]">Latest <span class="text-[#fb991c]">Articles</span></h2><p class="text-secondary">Diverse Range of articles related to html css and javascript</p></div><div class="grid gap-6 sm:grid-cols-2 md:grid-cols-3 pb-7"><divclass="flex transform flex-col gap-3 rounded-lg border p-3 transition-transform hover:scale-105"><figure class="relative h-40 w-full overflow-hidden bg-gray-200"><imgclass="absolute inset-0 h-full w-full rounded-md object-cover"src="https://plus.unsplash.com/premium_photo-1673984261110-d1d931e062c0?q=80&w=2070&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D"alt="demo"/></figure><a href="/react-tailwind-newsletter-component"><h3class="mb-2 text-xl font-bold text-[#161616] transition-colors duration-200 hover:text-[#fb991c]">React Tailwind Newsletter Component</h3><p class="text-gray-700">Learn how to build a responsive newsletter component using React and Tailwind CSS.</p><p class="mt-4 text-sm font-semibold text-[#161616]">14th July 2024 | 5 min read</p></a></div><divclass="flex transform flex-col gap-3 rounded-lg border p-3 transition-transform hover:scale-105"><figure class="relative h-40 w-full overflow-hidden bg-gray-200"><imgclass="absolute inset-0 h-full w-full rounded-md object-cover"src="https://images.unsplash.com/photo-1493612276216-ee3925520721?q=80&w=1964&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D"alt="demo"/></figure><a href="/advanced-javascript-concepts"><h3class="mb-2 text-xl font-bold text-[#161616] transition-colors duration-200 hover:text-[#fb991c]">Advanced JavaScript Concepts</h3><p class="text-gray-700">Deep dive into closures, prototypes, and asynchronous programming in JavaScript.</p><p class="mt-4 text-sm font-semibold text-[#161616]">25th May 2024 | 10 min read</p></a></div><divclass="flex transform flex-col gap-3 rounded-lg border p-3 transition-transform hover:scale-105"><figure class="relative h-40 w-full overflow-hidden bg-gray-200"><imgclass="absolute inset-0 h-full w-full rounded-md object-cover"src="https://plus.unsplash.com/premium_photo-1663100722417-6e36673fe0ed?q=80&w=2070&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D"alt="demo"/></figure><a href="/introduction-to-typescript"><h3class="mb-2 text-xl font-bold text-[#161616] transition-colors duration-200 hover:text-[#fb991c]">Introduction to TypeScript</h3><p class="text-gray-700">An introductory guide to TypeScript, covering the basics and helping you get startedwith typed JavaScript.</p><p class="mt-4 text-sm font-semibold text-[#161616]">10th February 2024 | 7 min read</p></a></div><divclass="flex transform flex-col gap-3 rounded-lg border p-3 transition-transform hover:scale-105"><figure class="relative h-40 w-full overflow-hidden bg-gray-200"><imgclass="absolute inset-0 h-full w-full rounded-md object-cover"src="https://images.unsplash.com/photo-1560732488-6b0df240254a?q=80&w=2070&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D"alt="demo"/></figure><a href="/building-a-rest-api-with-node-js"><h3class="mb-2 text-xl font-bold text-[#161616] transition-colors duration-200 hover:text-[#fb991c]">Building a REST API with Node.js</h3><p class="text-gray-700">Step-by-step guide to creating a RESTful API using Node.js and Express.</p><p class="mt-4 text-sm font-semibold text-[#161616]">15th April 2024 | 12 min read</p></a></div><divclass="flex transform flex-col gap-3 rounded-lg border p-3 transition-transform hover:scale-105"><figure class="relative h-40 w-full overflow-hidden bg-gray-200"><imgclass="absolute inset-0 h-full w-full rounded-md object-cover"src="https://images.unsplash.com/photo-1501594907352-04cda38ebc29?q=80&w=2070&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D"alt="demo"/></figure><a href="/css-grid-layout-complete-guide"><h3class="mb-2 text-xl font-bold text-[#161616] transition-colors duration-200 hover:text-[#fb991c]">CSS Grid Layout: A Complete Guide</h3><p class="text-gray-700">Master CSS Grid Layout with this comprehensive guide covering all the essentials andadvanced techniques.</p><p class="mt-4 text-sm font-semibold text-[#161616]">20th March 2024 | 15 min read</p></a></div><divclass="flex transform flex-col gap-3 rounded-lg border p-3 transition-transform hover:scale-105"><figure class="relative h-40 w-full overflow-hidden bg-gray-200"><imgclass="absolute inset-0 h-full w-full rounded-md object-cover"src="https://plus.unsplash.com/premium_photo-1680404114169-e254afa55a16?q=80&w=1964&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D"alt="demo"/></figure><a href="/getting-started-with-next-js"><h3class="mb-2 text-xl font-bold text-[#161616] transition-colors duration-200 hover:text-[#fb991c]">Getting Started with Next.js</h3><p class="text-gray-700">A beginner’s guide to building server-rendered React applications with Next.js.</p><p class="mt-4 text-sm font-semibold text-[#161616]">10th June 2024 | 8 min read</p></a></div></div></section></body></html>