On This Page
- # What is an Enum?
- # Why Use Enum in React with TypeScript?
- # When to use an enum in ReactJS
- # React Component without Enum
- # How to Create Enum in typescript
- # React Component with Enum
- # Benefits of using enum
- # Convert if statement to a switch statement in JSX
- # How to create enum in Javascript
- # Enum Best Practices in React
Enums in TypeScript are powerful tools to organize and maintain your codebase. In ReactJS projects, they can help you manage screen states, user roles, themes, or any other constant values that might otherwise become magic strings and lead to bugs.
In this article, we'll explore how to use enums in a practical React example to make your components safer, cleaner, and easier to maintain.
What is an Enum?
An enum
(short for enumeration) is a way to define a set of named constants.
You can use enums anywhere you'd otherwise use a string or number constant.
As per typescript docs definition:
Enums allow a developer to define a set of named constants. Using enums can make it easier to document intent, or create a set of distinct cases.
Here's a simple example:
enum Direction {Up = 'UP',Down = 'DOWN',Left = 'LEFT',Right = 'RIGHT',}
Why Use Enum in React with TypeScript?
Enums can be used for:
- Managing screen states in a multi-screen app
- Defining user roles (Admin, Guest, Member)
- Handling UI themes (Dark, Light, Auto)
- Mapping status codes (Pending, Completed, Failed)
They make your code more descriptive and refactor-friendly.
When to use an enum in ReactJS
We can use an enum for choices for certain things, like yes or no, screen types, etc.
For example, we want to build a quiz application in ReactJS.
As Quiz application screens or components are condition based, as soon the app loads, we show the loader first, then the home screen, then the quiz questions screen. When the user completes all questions, we show the result screen.
The screen sequence of a quiz app is:
- Loading Screen
- Home screen
- Quiz Screen
- Result Screen
We need to define a state to track on which screen we are and show the data.
React Component without Enum
First, we will build a component in plain ReactJS without the Typescript enum, so we can see the difference between them.
import { useState } from 'react'const App = () => {const [currentScreen, setCurrentScreen] = useState('homeScreen')return (<>{currentScreen === 'appLoader' && <p>Loading...</p>}{currentScreen === 'homeScreen' && <p>Please select the quiz type</p>}{currentScreen === 'questionScreen' && <p>First question...</p>}{currentScreen === 'resultScreen' && <p>You are passed</p>}</>)}export default App
We will render JSX
as per the current state. The first and foremost thing is all the screen names are hard-coded. We do not recommend and consider it as best practice. In the plain react component, we have to check the screen names while typing so we don’t make any spelling mistakes.
In short,
- Harder to refactor
- No autocomplete or intellisense
- String values can easily have spelling mistakes or typos.
Typescript enum will solve this problem.
How to Create Enum in typescript
Enum is an special class and can be defined using enum
keyword:
enum ScreenTypes {AppLoader = 'appLoader',HomeScreen = 'homeScreen',QuestionScreen = 'questionScreen',ResultScreen = 'resultScreen',}
React Component with Enum
Now we have transformed the above react component from javascript to typescript file.
import { useState } from 'react'export enum ScreenTypes {AppLoader = 'appLoader',HomeScreen = 'homeScreen',QuestionScreen = 'questionScreen',ResultScreen = 'resultScreen',}const App = () => {const [currentScreen, setCurrentScreen] = useState(ScreenTypes.ResultScreen)return (<p>{currentScreen === ScreenTypes.AppLoader && <p>Loading...</p>}{currentScreen === ScreenTypes.HomeScreen && <p>Please select the quiz type</p>}{currentScreen === ScreenTypes.QuestionScreen && <p>First question...</p>}{currentScreen === ScreenTypes.ResultScreen && <p>You are passed</p>}</>)}export default App
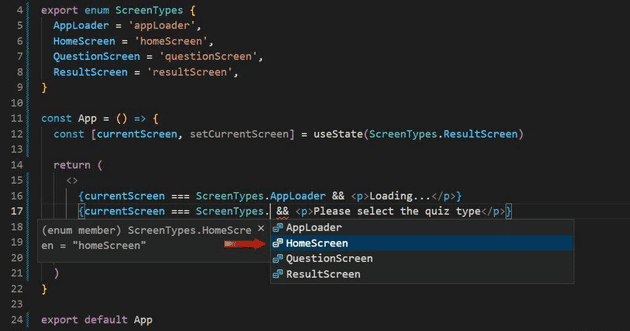
Benefits of using enum
- We don’t have to remember or look back to see available choices or options, we will get IntelliSense for that as you can see in above image.
- Since we are getting IntelliSense so there is very less chance of spelling mistakes or typos.
We are not yet done, it's better to change this component to switch statements, instead of multiple if conditions, it's the best practice to use the switch cases.
Convert if statement to a switch statement in JSX
If we have 4 or above conditions it's better to change it into switch statements for better readability and it's a best practice. We are going to change above component from multiple if statements to switch cases.
import { useState } from 'react'export enum ScreenTypes {AppLoader = 'appLoader',HomeScreen = 'homeScreen',QuestionScreen = 'questionScreen',ResultScreen = 'resultScreen',}const App = () => {const [currentScreen, setCurrentScreen] = useState(ScreenTypes.HomeScreen)switch (currentScreen) {case ScreenTypes.AppLoader:return <p>Loading...</p>case ScreenTypes.HomeScreen:return <p>Please select the quiz type</p>case ScreenTypes.QuestionScreen:return <p>First question...</p>case ScreenTypes.ResultScreen:return <p>You are passed</p>default:return <p>Loading</p>}}export default App
How to create enum in Javascript
It's good to know that we can also mimic enum in javascript using Object.freeze()
method.
Object.freeze()
means object is frozen, we can’t change any of object properties by any mistake.
const screens = Object.freeze({AppLoader: 'appLoader',HomeScreen: 'homeScreen',QuestionScreen: 'questionScreen',ResultScreen: 'resultScreen',})
Enum Best Practices in React
- Use string enums for readability
- Keep enums in their own files (
ScreenType.ts
) - Use PascalCase for enum names
- Don’t overuse enums, use union types when appropriate
- Prefer enums for related constant values reused across multiple components
We are done with enum in ReactJS component. I hope you learned something new from this article. Don’t forget to share it with fellow developers.