On This Page
This React Typescript Tasklist/Todo app challenge requires you to perform CRUD operations with instructions to write clean, reusable, manageable, and scalable code.
CRUD refers to create, read, update, and delete. Mastering these operations in Reactjs means you can build any application.
This challenge is different and more challenging than the last three challenges you have completed. If you can complete this ReactJS Typescript Tasklist challenge independently, you can be easily hired as an intern/junior React developer.
This is not just a challenge, but my 3 years of working experience. The tasks you will be performing in this challenge are what a React developer performs daily. So, let's dive in and improve your React development skills!
How To Start Typescript Tasklist App
If you have decided to take this challenge, so here are the steps to start this ReactJS challenge:
- Fork Reactjs Coding Challenges GitHub repo
- Go to
task-list
folder usingcd task-list
via terminal - Run npm install or yarn to install dependencies
- Run the react server using
npm start
, you will then see the below screen on your local host
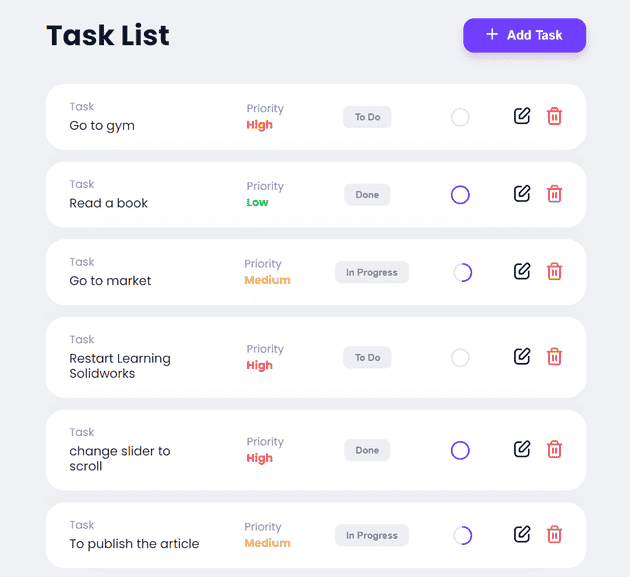
User Stories
There are different tasks you have to implement in this challenge. Follow these user stories step by step to avoid missing any functionality.
User Story 01: Add/Create a new task
If you click the add modal button, the add modal will pop up, where you will add a new task in input and select a priority (low, medium, high). Here’s what you should do while creating a new task:
- After clicking add task button, the task will be added to the UI.
- By default, the new task status will be "To Do," and progress will be 0 (empty).
- Each task will have a unique "Id."
- User can not add an empty task (or task with empty spaces), and If task input is empty, add or edit button will be disabled.
- Newly added tasks will appear on top of all tasks.
- Selected task priority button will look like below the "Add Task Form" image. You can also play with the demo app app.
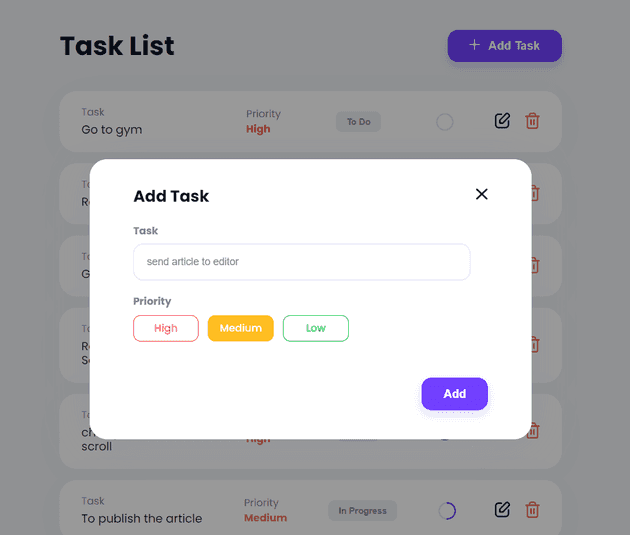
User Story 02: Delete a task
The delete modal will pop up when you click the delete icon. If you click the delete button, the particular task will be deleted from UI, and the modal will close. If you click the cancel button, only the modal will be closed.
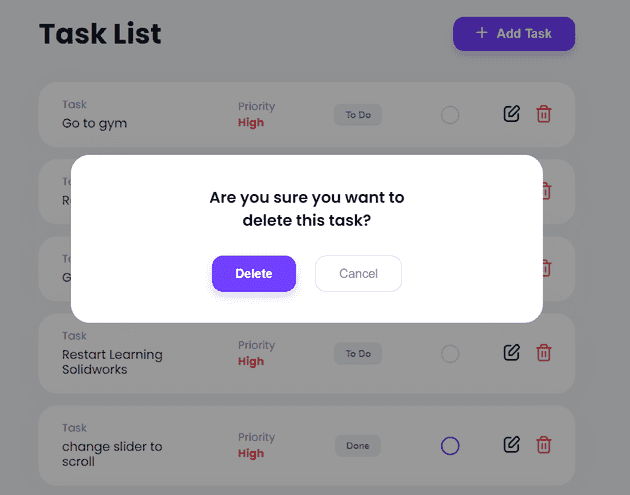
User Story 03: Edit/Update a Task
-
When you click the edit icon, the modal will pop up, with that particular task information filled. Play with the demo app app for better clarity
-
When the user edits the task and presses the Edit button, it will replace that task with a newly added task
-
User can change the task status by clicking the status (To Do, In Progress, Done) button.
a. If the task status is "Todo", progress will be 0 (empty circular progress).
b. If the status is "In Progress," circular progress will be 50% (half-filled).
c. If the status is "Done," the circular progress bar will be completely filled (100%).
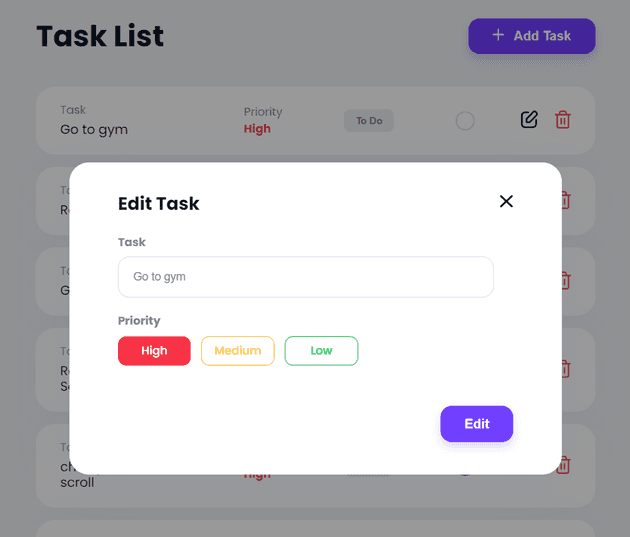
Further Instructions
- Use the same form for add and edit tasks (handle add and edit feature conditionally).
- Since this project is for beginner React developers so I won't recommend using context or redux for state management.
- Write all typescript types.
- Make sure there isn't any error in the console.
UI and Features Suggestions for React Typescript Todo Application
Once you have completed the challenge, you can move ahead and make it better by adding below features:
-
Build a Nodejs API for it and update it to MERN App
-
You can turn this one-page task list into a dashboard where users can filter tasks on the basis of status, priority, and tags
-
You can also add a tag for each task. Eg. project, freelance, grocery, office, etc
Hint and Resources
- How to update an array of object article will help you with the edit task.
- You can keep track of (the current form) state to render, add, and edit form conditionally.
- To delete a task, get the task's id and filter out that task using the Javascript
filter()
method. - Fullstack React with TypeScript is the complete guide to using TypeScript with React. Learn TypeScript patterns with React additional ecosystem advice (testing, redux, SSR) by building several apps including a Trello clone, a Medium-like website, testing with a digital-item e-comm app, and more!
Final Result
The final functioning Typescript Tasklist App will look like this.
Working Solution For Typescript Tasklist App
For suggestions and collaboration, you can send me an email at [email protected] or can reach out to me on LinkedIn or Twitter.
Important!
You can also subscribe to Codevertiser Magazine Newsletter and get the latest coding challenges delivered straight to your inbox