On This Page
- # What Is Loading Spinner Button In ReactJS?
- # Why Use And Create Loading Spinner Button In ReactJS?
- # When To Implement Loading Spinner Button In ReactJS?
- # How Can You Create Loading Spinner Button In ReactJS?
- # Step#1: Create Basic UI Button Structure
- # Step#2: Loading the Text Button Display
- # Step#3: Button Loading Spinner
- # Step#4: Disabling Button While Loading
- # Step#5: Coding Logic to Create Loading Spinner Button in ReactJS
- # Complete Code to Create Loading Spinner Button in ReactJS
- # App.js
- # CSS
- # Codepen Demo | Loading Spinner Button
As an experienced ReactJS developer, I focus not only on the functionality of the app, but also code to improve its UI/UX experience. Why? Because this factor can greatly influence the engagement of your target users with the app. And for any app’s success, it’s important to have a pleasant UX for almost all users to become the leader in the digital market.
Since we are talking about the UX of the app, then adding a loading spinner button to your app design can prominently get you extraordinary results in terms of user engagement. You can keep your users hooked to the app during the response time. In fact, it’s a nice way of letting your users know that the server is processing their request, so they must wait for the response.
To understand more about loading spinner button in ReactJS, I’ll be discussing the following points in this tutorial:
-
What is loading spinner button in ReactJS?
-
Why use and create loading spinner button in ReactJS?
-
When to implement loading spinner button in ReactJS?
-
How can you create loading spinner button in ReactJS?
Without any further ado, let’s get started!
What Is Loading Spinner Button In ReactJS?
The loading spinner button is simply a ReactJS component that lets you show an ongoing process. The event triggers when the user clicks a button. The request is then sent to the server-side for processing, while during the event it changes the button into a loading button with a spinner. It gets back to the user with the output and changes the button back to its original appearance – which has no spinner but button text.
The output of this process can simply be some data display, validation of some information, or any action that is coded on that particular button. You may even have seen such loading spinner buttons on various apps, such as add/delete, submit, login, or similar other buttons turning into loading spinner buttons upon your click.
Why Use And Create Loading Spinner Button In ReactJS?
The buttons that require some time to process any request submitted from the client-side to the server-side can use this button to inform the users about the request progress. The developers use this ReactJS component to let the users know that they should wait for the response from the app as their current submitted request is being processed at the server end.
To understand the concept of loading spinner button in ReactJS more thoroughly, let’s assume a sign-in form with a login button. When you click the login button, this sends the request to the server-side and performs the coded action on the provided input within its database.
During this waiting period, the button changes to a loading button with a spinner display and prevents sending another request from the user end to the server side until the current request is completely processed. I hope you can understand the basic concept of the loading spinner button in ReactJS with the above mentioned example. But if you are still confused about it, then you can learn more in the further section of the tutorial with the practical implementation of this ReactJS component.
When To Implement Loading Spinner Button In ReactJS?
Besides using the loading button with a spinner display as an interactive animation during the response time from the server to the app, you must also know when to use this ReactJS component. So, here are a few things to ask yourself when you want to create loading spinner button in ReactJS for any of your apps:
- Does this button improve the user interface (UI) and user experience (UX) of your ReactJS app?
- Does this button help your user understand that there is an ongoing process in the background and they must wait during the response time?
- Does this button add any valuable contribution to your ReactJS app for your target users? If the answer to these questions is YES, then it’s a safe bet to add a nice loading spinner button to your ReactJS app.
How Can You Create Loading Spinner Button In ReactJS?
With all the necessary information at hand, you can now create the button with a spinner display in ReactJS for your app. So, it's time to write the code!
Step#1: Create Basic UI Button Structure
First, we will build the UI for the simple button, just as shown below:
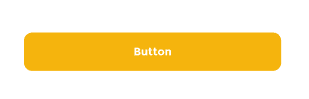
To develop the UI of the button, we’ll write the following code:
const Button = ({ onSubmit, text }) => {return (<button className="submit-btn" onSubmit={onSubmit}>{text}</button>)}export default Button
Step#2: Loading the Text Button Display
As of now, we’ll show the text inside the button and pass it via props
from the parent. We’ll write a conditional if statement for the loader. So, if the loading prop is true
, show the loader otherwise display the text
on the button.
const Button = ({ onSubmit, text, loading }) => {return (<button className="submit-btn" onSubmit={onSubmit}>{!loading ? text : 'loading...'}</button>)}export default Button
Step#3: Button Loading Spinner
In this step, we want to show the spinner instead of showing the loading text. And to do that, we are going to use spinner SVG as shown in the code snippet below:
<svg width="13" height="14" viewBox="0 0 13 14" fill="none" xmlns="http://www.w3.org/2000/svg"><pathd="M4.38798 12.616C3.36313 12.2306 2.46328 11.5721 1.78592 10.7118C1.10856 9.85153 0.679515 8.82231 0.545268 7.73564C0.411022 6.64897 0.576691 5.54628 1.02433 4.54704C1.47197 3.54779 2.1845 2.69009 3.08475 2.06684C3.98499 1.4436 5.03862 1.07858 6.13148 1.01133C7.22435 0.944078 8.31478 1.17716 9.28464 1.68533C10.2545 2.19349 11.0668 2.95736 11.6336 3.89419C12.2004 4.83101 12.5 5.90507 12.5 7"stroke="white"/></svg>
After the Spinner SVG part is done, we’ll import the loading spinner from the assets folder of the codebase by using the following code:
import { ReactComponent as Loader } from '../../assets/icons/loader.svg'const Button = ({ onSubmit, text, loading }) => {return (<button className="submit-btn" onClick={onSubmit}>{!loading ? text : <Loader className="spinner" />}</button>)}export default Button
To get the continuous effect of the loading spinner button in ReactJS, we’ll need to add a spinner class and use CSS keyframes
. And this can be done with the code written below:
.spinner {animation-name: spin;animation-duration: 500ms;animation-iteration-count: infinite;animation-timing-function: linear;}@keyframes spin {from {transform: rotate(0deg);}to {transform: rotate(360deg);}}
Step#4: Disabling Button While Loading
Now, we are left with the coding part that disables the button during the response time to the user end. We’ll use the button tag’s disabled
props, as it can make the button disable (or unclickable) during the response time. You can write the code below:
const Button = ({ onSubmit, text, loading, disabled }) => {return (<button className="submit-btn" onClick={onSubmit} disabled={disabled}>....</button>)}export default Button
To create loading button with a spinner in a more professional display, we have also added some styling for the disabled button. We have changed the background and text color of the button that makes it prominently look different from its original appearance using the code below:
.submit-btn:disabled {background: #e7e8e9;color: #9fa3a9;cursor: not-allowed;}
So, this is how the disabled button with a spinner display will look like:
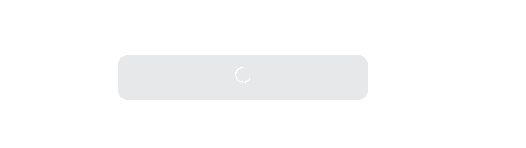
Till this point, we are done with the UI part of the loading spinner button. Now, we’ll move to the next most important part of the tutorial which addresses the coding logic of the loading spinner button.
Step#5: Coding Logic to Create Loading Spinner Button in ReactJS
After we are done with the UI part, it's time to write the coding logic to create loading spinner button in ReactJS.
In real apps we show the loader between request and response, to mimic the functionality we need to define a state
to keep track if loading is true
or not.
import { useState } from 'react'import Button from './components/LoadingButton/Button'const App = () => {const [showLoader, setShowLoader] = useState(false)const onSubmit = () => {console.log('button clicked')}return <Button text="Submit" onSubmit={onSubmit} loading={showLoader} disabled={showLoader} />}export default App
With the above coding, we’ll make the loader state (showLoader)
to true
status for 1 second using the setTimeout
function when the user clicks the button. So, after one second the loading will revert to false
status.
Remember
In real-world apps, we don’t implement this practice. We show the loader or the loading spinner button in ReactJS during the API request session.
Since our loading button is a separate isolated component to use in multiple places on the app, we are rendering it inside the App
(parent) component. Here is a code for it:
const App = () => {const [showLoader, setShowLoader] = useState(false)const onSubmit = () => {setShowLoader(true)setTimeout(() => setShowLoader(false), 1000)}return (<Button.../>)}export default App
If you are new to React or struggling to learn React, you may find this comprehensive ReactJS course helpful.
Complete Code to Create Loading Spinner Button in ReactJS
Below is the complete source code that we have used to create loading spinner button in ReactJS in this tutorial:
import { ReactComponent as Loader } from '../../assets/icons/loader.svg'const Button = ({ onSubmit, text, loading = false, disabled }) => {return (<button className="submit-btn" onClick={onSubmit} disabled={disabled}>{!loading ? text : <Loader className="spinner" />}</button>)}export default Button
App.js
import { useState } from 'react'import './App.css'import Button from './components/LoadingButton/Button'const App = () => {const [showLoader, setShowLoader] = useState(false)const onSubmit = () => {setShowLoader(true)setTimeout(() => setShowLoader(false), 1000)}return <Button text="Submit" onSubmit={onSubmit} loading={showLoader} disabled={showLoader} />}export default App
CSS
@import url('https://fonts.googleapis.com/css2?family=Anek+Malayalam:wght@100;200;300;400;500;600;700;800&display=swap');.submit-btn {font-family: 'Anek Malayalam', sans-serif;background: #f5b40d;border: none;outline: none;border-radius: 8px;font-weight: 700;font-size: 14px;color: #ffffff;min-width: 200px;min-height: 36px;cursor: pointer;outline: none;}.submit-btn:disabled {background: #e7e8e9;color: #9fa3a9;cursor: not-allowed;}.spinner {animation-name: spin;animation-duration: 500ms;animation-iteration-count: infinite;animation-timing-function: linear;}@keyframes spin {from {transform: rotate(0deg);}to {transform: rotate(360deg);}}
Codepen Demo | Loading Spinner Button
I hope this tutorial helped you create loading spinner button in ReactJS with no difficulty at all. But still, if you have any queries, please feel free to reach out to me. Happy Coding!